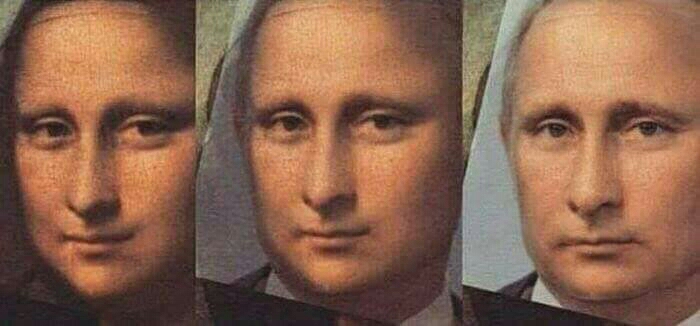
We are going to deploy our application to wildfly to cloud server, while we need to set test environment and production environment. It’s complecated to set up double server with different ports, because it means doing the same deployment things twice. One simple way is to deploy the same app in the same wildfly, but with different app names. To achieve this, you can simply rename app.war
to newname.war
. So the context becomes to newname
. You can visit web application through http://localhost:8080/newname
. You need to do this each time you do deploy. There’s another elegant way to achieve this. Add jboss-web.xml
to WEB-INF
and package it to the war. jboss-web.xml
looks like this:
1 |
|
And if the war
contains resource connecting like database, file system. You probably need to connect to different databases, for databases, we usually put data source in META-INF/persistence.xml
, like this:
1 | <jta-data-source>java:jboss/datasources/DS</jta-data-source> |
I can’t change it each time do deploy. It’ll be good if the context-root
and jta-data-source
can be changed during packaging. Maven profile works like a charm.
I have to attach document because it explains more details and more complemental. When we looking for solutions we usually google it instead of reading docs, because if we find the right place, it will solve our issue directly, but we might be blind for why and how or some other usages. Reading docs might take much more time and probably you lost in it. It depends on situations. It’s up to you.
Back to maven profile, it’s like “pom.xml settings”, during packing, you can choose which profile to use. And packaging the application for you. In this case, we use profile to set property with different values. Remember this, profile can do more than set property values, it can use different jdks to compile, copy different resource files, package for different hosts, etc. I make example for data source. First, create a property in <properties>
element.
1 | <properties> |
Then create profiles
1 | <profiles> |
Notice <jta-data-source>
, <final-artifactId>
, they are defined in <profile>
element, belongs to each profile, so they can be different values. And in <finalName>
, we use final-artifactId
and project.version
to generate package name. It will be like “mvn-profile-1.0-SNAPSHOT”, “mvn-profile-dev-1.0-SNAPSHOT”. All properties can be used by ${propertyname}
. Properties can be used out side of pom.xml
. When packaing, maven can filter files and replace ${propertyname}
with real value. For example, if we want to change context-root
of jboss-web.xml
. We can change it like:
1 | <context-root>${final-artifactId}</context-root> |
Besides, we need to set filter in pom.xml
in <build><plugins><plugin>
element:
1 | <plugin> |
maven-war-plugin will collect dependencies, resources and packing the files. Noticing element <filtering>
, default is false. It will filter the files and replacing properties. But you should be responsible for files to be filtered. You just include the files that need to be filtered. Other files should be copy no filtering. Because image, certification would be broken after filtering.
All codes can be found here. After downloading the module, you can verify this by runningmvn clean package
and mvn clean package -P dev
to see the differences. Or you can download the packages here.